Plan
Do it standing, about same mix of slides and coding as before, handouts.
Slide: Tom Waits + install
Slide/Code: fire up scala and show off syntax (REPL)
Slide: motivation
Slide: My verdict + References
Slide/Code: Bonus section
Presentation
“Your hands are like dogs, going to the same places they’ve been. You have to be careful when playing is no longer in the mind but in the fingers, going to happy places. You have to break them of their habits or you don’t explore; you only play what is confident and pleasing. I’m learning to break those habits by playing instruments I know absolutely nothing about, like a bassoon or a waterphone.”—Tom Waits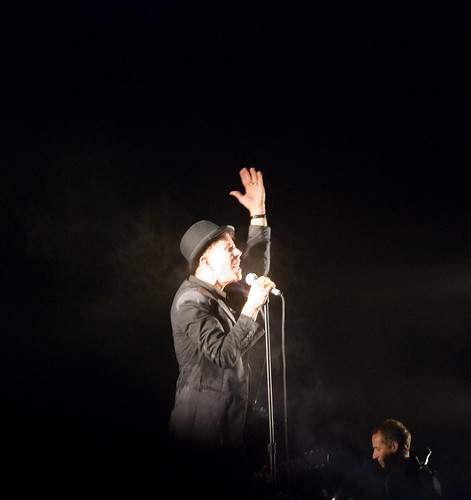
Install
Download zip-file, unzip, set SCALA_HOME and add to PATH.Then: install plugins for NetBeans (6.7+, prefer 6.8) and eclipse (3.5+).
I did best letting NetBeans handle project (not Maven).
Syntax / REPL
scala.bat: Quick Read Evaluate Print LoopCode | Points
to make |
class PhoneNumber( val kind: String, var no: String ) { |
switch to name: type
val / var / def primary constructor Concise |
object PhoneNumber{ |
object, no statics
apply is "default method" |
val phonebook = Map( |
Expressive |
println( phonebook("Tommy mobil" ).no ) | Concise |
phonebook.foreach(tuple => println( tuple._1 + ":" + tuple._2 )) | High-level
functional iteration |
1.1.+(2.2)
1 until 10 |
Pure OO
operator mode for methods |
val xmlE = <a href="index.html"> {3 + 4} </a> |
Pragmatic |
.
Motivation
Evolution, not revolution: Java-like, interoperates with java (seamlessly). - can extend java classes
- can implement java interfaces
- understands java generics
- uses java primitives
Easy to do easy stuff, AND facilitates the hard stuff.
More up2date language - builds on java lessons, adds:
- Facilitates DSL with several short-hand notations
- Traits (~multiple inheritance)
- ++
Pragmatic
"I can honestly say if someone had shown me the Programming Scala book by by Martin Odersky, Lex Spoon & Bill Venners back in 2003 I’d probably have never created Groovy." — James Strachan
"If I were to pick a language to use today other than java, it would be Scala" — James Gosling (from before he left Oracle)
My verdict: lead me into...
Wonderful way of coming from Java to Functional programming. I started out writing java-like OO, but wolfed out by the end of my second 3-hour session.
The book fuelled this transformation.
Scala feels very good so far, I now like Java less...
References
Scala site.
Book "Programming in Scala" (Odersky++) is very good for learning, not so for reference.
The busy Java developer's guide to Scala: articles on DeveloperWorks
From JavaZone 09:
Bonus section
Start with Java Discovery and refactor a suitable class:- common.ServiceDescription (for properties and basics) NB! keep scala-code for equals / canEqual
Code | Points to make |
TestServer | object and main |
client.v2.Notifier | operators |
client.ServiceBrowser:
| traits
Function is first-class object Option + match |
sclanb.Main.recurse2 | Closures, and how crazy I got |
sclanb.Main |
|
client.ServiceBrowserListener | abstract, no interface |
FirstSwingApp. | DSL, Swing |
Code from ch30 | Actors |